Understanding the Aggregator Pattern in Microservices Development
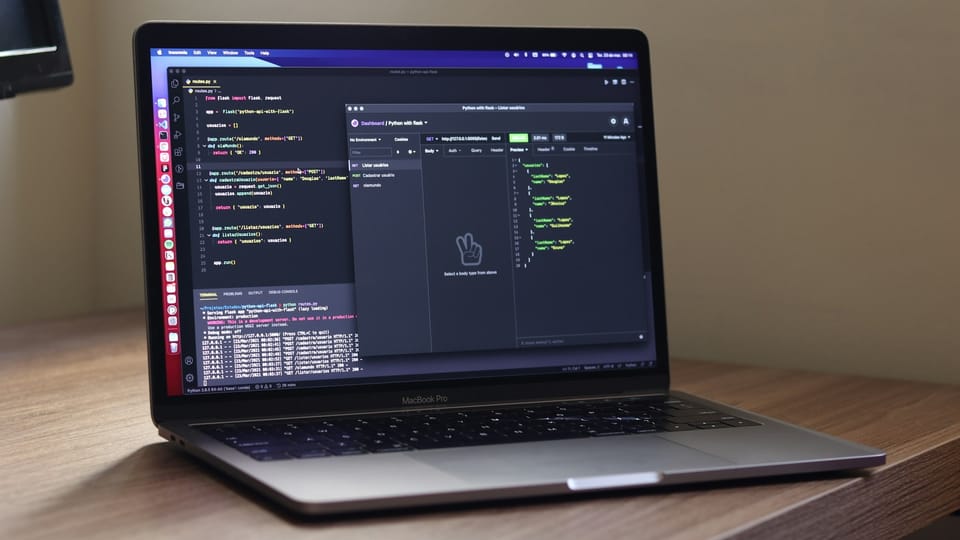
The Aggregator Pattern is a critical design pattern in microservices architecture, especially when dealing with complex systems where different microservices must collaborate to fulfill a single request. In this blog post, we will dive into the Aggregator Pattern and how it works. We will explore its implementation using .NET, with code snippets to help you understand the concept better and some of the best practices and considerations for effectively using this pattern in your microservices architecture.
What is the Aggregator Pattern?
In a microservices architecture, services are often fine-grained, which means that a single request from a client may require data from multiple microservices. The Aggregator Pattern provides a way to aggregate the results from multiple microservices into a single response, which can then be returned to the client.
The pattern involves creating an aggregator service that orchestrates the calls to the necessary microservices, collects their responses, and compiles them into a unified response. This pattern is beneficial when the client needs data from several sources but does not need to know where the data comes from.
When to Use the Aggregator Pattern
The Aggregator Pattern is most effective in the following scenarios:
- Complex Client Requests: When a client request requires data from multiple microservices and combining these results into a single response is necessary.
- Performance Optimization: When you want to reduce the number of client-server interactions by consolidating multiple service calls into a single call.
- Simplifying Client Logic: When you want to abstract the complexity of multiple service calls from the client, simplify the client-side code.
Implementing the Aggregator Pattern in .NET
Let’s walk through an example of implementing the Aggregator Pattern in a .NET microservices architecture.
Example Scenario
Imagine we have a microservices-based e-commerce application. The application has the following microservices:
- Product Service: Provides details about products.
- Inventory Service: Provides information about product stock levels.
- Pricing Service: Provides pricing details for products.
We want to create an aggregator service that provides a consolidated view of a product, including its details, stock availability, and pricing.
Step 1: Creating the Aggregator Service
The aggregator service will orchestrate calls to the three microservices and return a combined response to the client.
public class ProductAggregatorService
{
private readonly HttpClient _httpClient;
public ProductAggregatorService(HttpClient httpClient)
{
_httpClient = httpClient;
}
public async Task<ProductDetailsDto> GetProductDetailsAsync(int productId)
{
var productTask = _httpClient.GetStringAsync($"https://productservice/api/products/{productId}");
var inventoryTask = _httpClient.GetStringAsync($"https://inventoryservice/api/inventory/{productId}");
var pricingTask = _httpClient.GetStringAsync($"https://pricingservice/api/pricing/{productId}");
await Task.WhenAll(productTask, inventoryTask, pricingTask);
var product = JsonSerializer.Deserialize<ProductDto>(await productTask);
var inventory = JsonSerializer.Deserialize<InventoryDto>(await inventoryTask);
var pricing = JsonSerializer.Deserialize<PricingDto>(await pricingTask);
return new ProductDetailsDto
{
Product = product,
Inventory = inventory,
Pricing = pricing
};
}
}
Step 2: Creating DTOs
Define the Data Transfer Objects (DTOs) that will be used to hold the data fetched from the microservices.
public class ProductDto
{
public int Id { get; set; }
public string Name { get; set; }
public string Description { get; set; }
}
public class InventoryDto
{
public int ProductId { get; set; }
public int StockLevel { get; set; }
}
public class PricingDto
{
public int ProductId { get; set; }
public decimal Price { get; set; }
}
public class ProductDetailsDto
{
public ProductDto Product { get; set; }
public InventoryDto Inventory { get; set; }
public PricingDto Pricing { get; set; }
}
Step 3: Handling API Responses and Aggregation
In this step, the responses from the different services are aggregated into a single ProductDetailsDto
object that will be returned to the client.
public class ProductDetailsDto
{
public ProductDto Product { get; set; }
public InventoryDto Inventory { get; set; }
public PricingDto Pricing { get; set; }
}
Best Practices for the Aggregator Pattern
- Error Handling: Ensure that your aggregator service gracefully handles errors. For example, if one service fails, decide whether to fail the entire request or return partial data.
- Caching: To improve performance, consider caching the responses from the microservices, mainly if the data stays mostly the same.
- Asynchronous Communication: Use asynchronous calls to prevent the aggregator service from blocking while waiting for other services to respond.
- Load Balancing: Use load balancing strategies to distribute the load evenly across the services.
Conclusion
The Aggregator Pattern is a powerful tool in microservices architecture, allowing you to create a unified API with which clients can interact easily. It simplifies the client-side logic by abstracting the complexity of multiple service calls and helps improve performance by reducing the number of network hops.
For a more in-depth understanding and additional design patterns in microservices, I highly recommend getting a copy of Microservices Design Patterns in .NET by Trevoir Williams. This book is a valuable resource that dives deeper into various patterns and best practices for building robust microservices architectures using .NET. You can purchase it from this link and take your microservices development to the next level.
Member discussion