Understanding Objects and How They Are Created in .NET
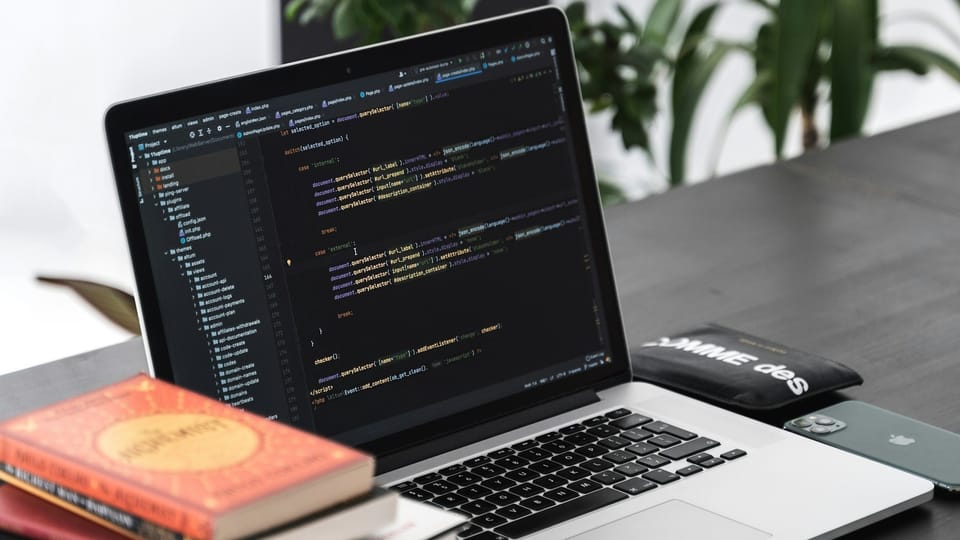
Understanding how objects are created and managed in .NET programming is fundamental to writing efficient and effective code. Objects are the backbone of object-oriented programming (OOP), and in .NET, their creation and lifecycle management are closely tied to the runtime environment, specifically the Common Language Runtime (CLR). In this article, we'll explore the intricacies of object creation in .NET, delving into how objects are instantiated, stored, and managed in memory.
What is an Object?
An object in .NET is an instance of a class, which is a blueprint defining that object's properties, methods, and behaviors. When you create an object, you're essentially allocating a block of memory that can store the data defined by the class and allow access to the methods provided by that class.
Here’s a simple example of a class and how an object of that class is created:
public class Person
{
public string FirstName { get; set; }
public string LastName { get; set; }
public void Introduce()
{
Console.WriteLine($"Hello, my name is {FirstName} {LastName}");
}
}
// Creating an object of the Person class
Person person = new Person();
person.FirstName = "John";
person.LastName = "Doe";
person.Introduce();
In this example, Person
is a class with two properties (FirstName
and LastName
) and a method (Introduce
). The line Person person = new Person();
creates an instance of the Person
class, also known as an object. This object now resides in memory and can be interacted with using its properties and methods.
Object Creation and Memory Allocation
When an object is created in .NET, the CLR handles memory allocation through a process that involves the stack and heap. These two memory areas play different roles in storing and managing objects.
- Stack: The stack is used for storing value types and references to objects. It's a Last-In-First-Out (LIFO) structure where method calls, local variables and reference pointers are stored.
- Heap: The heap stores objects, particularly reference types like classes. When an object is created, memory is allocated on the heap, and a reference to that memory location is stored on the stack.
Consider the following example:
public class Car
{
public string Make { get; set; }
public string Model { get; set; }
}
// Creating an object of the Car class
Car car = new Car();
car.Make = "Toyota";
car.Model = "Corolla";
In this case, the Car
object is created on the heap, and a reference to this object is stored on the stack. The car
variable on the stack points to the actual Car
object on the heap. This distinction is crucial because it explains how .NET manages memory and why particular objects behave the way they do.
Stack vs. Heap in Object Creation
Differentiating between the stack and heap is vital for understanding how objects are created and managed. Here’s a quick summary:
- Stack Allocation: Value types (
int
,float
,struct
) are stored directly on the stack. When a method is called, space is reserved on the stack for all the method's local variables. Once the method execution is complete, these variables are removed from the stack. - Heap Allocation: Reference types (like classes) are allocated on the heap. This memory is managed by the Garbage Collector (GC), which periodically cleans up objects that are no longer referenced.
Here's how it looks in code:
int x = 10; // Value type stored on the stack
Car car = new Car(); // Reference type stored on the heap
The Role of the Garbage Collector in Object Lifecycle
Once an object is created, it stays in memory as long as it is in use. The CLR uses the Garbage Collector (GC) to clean up objects that no longer need cleaning. The GC periodically checks for objects no longer referenced and reclaims their memory. This process helps prevent memory leaks and ensures efficient use of memory.
The following conditions trigger garbage collection:
- The system has low physical memory.
- The heap's memory consumption exceeds a certain threshold.
- The
GC.Collect()
method is explicitly called (though this is generally not recommended).
Conclusion
Understanding how objects are created and managed in .NET is essential for writing efficient, reliable code. From the basics of class instantiation to the complexities of memory management, knowing how the CLR handles object creation can significantly improve your application’s performance and stability.
Whether you're a beginner or an experienced developer, mastering these concepts will help you write better, more optimized .NET applications. For a deeper dive into these topics, including best practices and advanced techniques, check out Effective .NET Memory Management by Trevoir Williams.
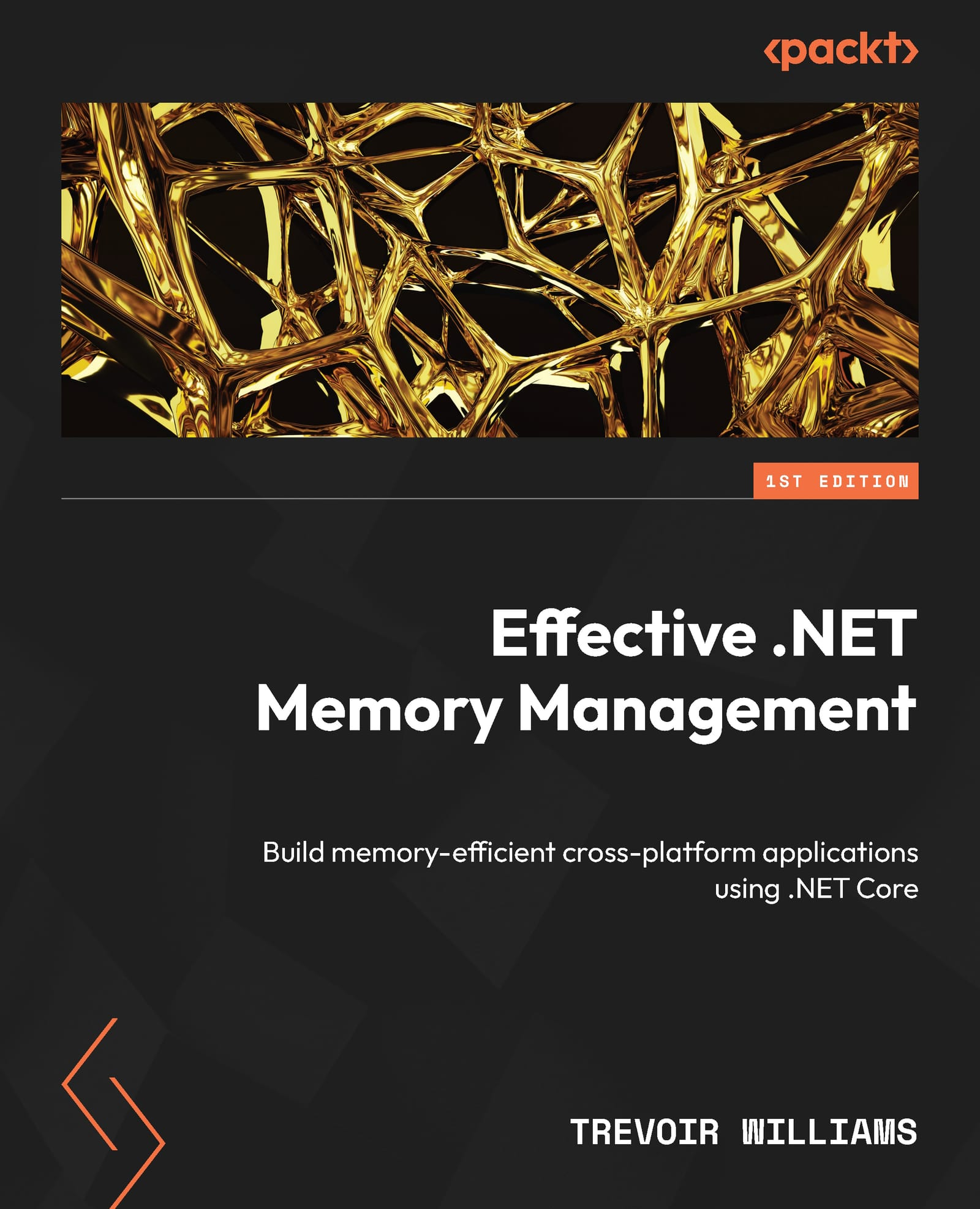
Member discussion