Understanding Memory Management in .NET
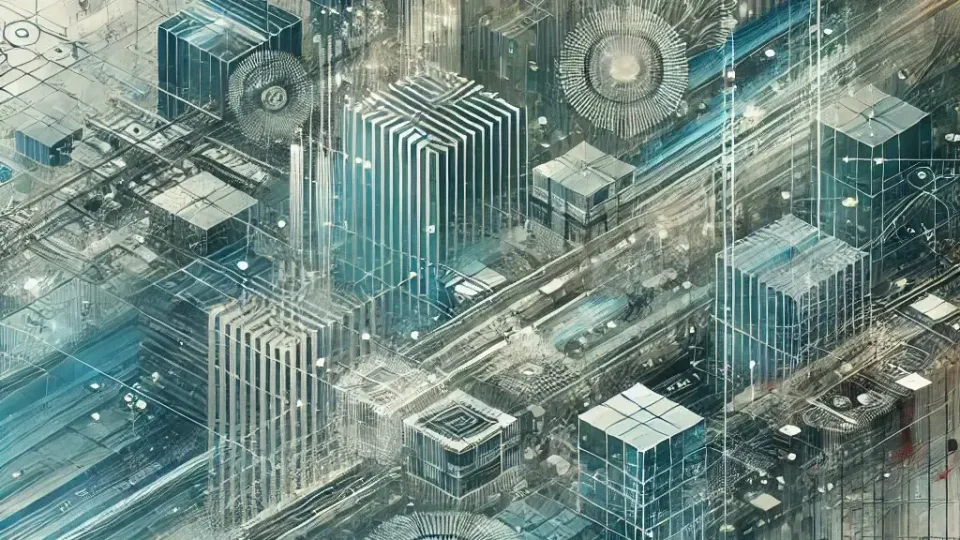
Memory management is a critical aspect of software development that can significantly impact the performance and reliability of your applications. In .NET programming, effective memory management ensures your applications run smoothly and efficiently, reducing the risk of memory leaks, fragmentation, and other performance bottlenecks.
At the heart of .NET's memory management is the Common Language Runtime (CLR), which provides a robust environment for automatic memory management. This means developers don't need to manually manage memory allocation and deallocation, as the CLR and its Garbage Collector (GC) handle these tasks.
Key Concepts of Memory Management in .NET
1. Garbage Collection: The Garbage Collector in .NET automatically reclaims memory that is no longer used by objects, freeing up resources and improving application performance. The GC operates on a generational model, with objects categorized into three generations based on their lifespan.
2. Memory Allocation: .NET manages memory allocation efficiently using the managed heap. Objects are allocated memory on the heap, and the CLR ensures that this allocation is contiguous for better performance. The process of allocating memory is streamlined, reducing the overhead that might occur with manual memory management.
3. Memory Leaks and Resource Management: Even with automatic garbage collection, developers must be vigilant about memory leaks. These occur when no longer needed objects are not correctly marked for collection, leading to wasted memory. Proper resource management, including using the IDisposable interface and the using statement, can help mitigate these issues.
4. Performance Considerations: Effective memory management directly impacts application performance. Poor memory management can lead to increased garbage collection cycles, which can prevent your application from becoming unresponsive or sluggish. Understanding how to optimize memory usage is crucial, especially in multi-threaded or resource-intensive applications.
Best Practices for .NET Memory Management
Use Appropriate Data Structures: Choose suitable data structures for your application. For example, replacing classes with structs where appropriate can reduce memory overhead, as structs are value types allocated on the stack rather than the heap.
Minimize Memory Footprint: Pre-size data structures when possible to avoid frequent memory reallocation. This helps reduce fragmentation and improve access times.
Profile and Optimize: Regularly profile your application to identify memory usage patterns and optimize accordingly. I can tell you that Visual Studio's diagnostic tools can be invaluable.
Avoid Large Object Allocations: Large objects are allocated on the Large Object Heap (LOH) and can lead to fragmentation if not appropriately managed. Consider breaking down large objects into smaller ones to avoid this issue.
Conclusion
Mastering memory management in .NET is essential for building high-performance, reliable applications. By understanding the intricacies of the CLR, garbage collection, and best practices in memory management, you can optimize your applications to run more efficiently and avoid common pitfalls like memory leaks and fragmentation.
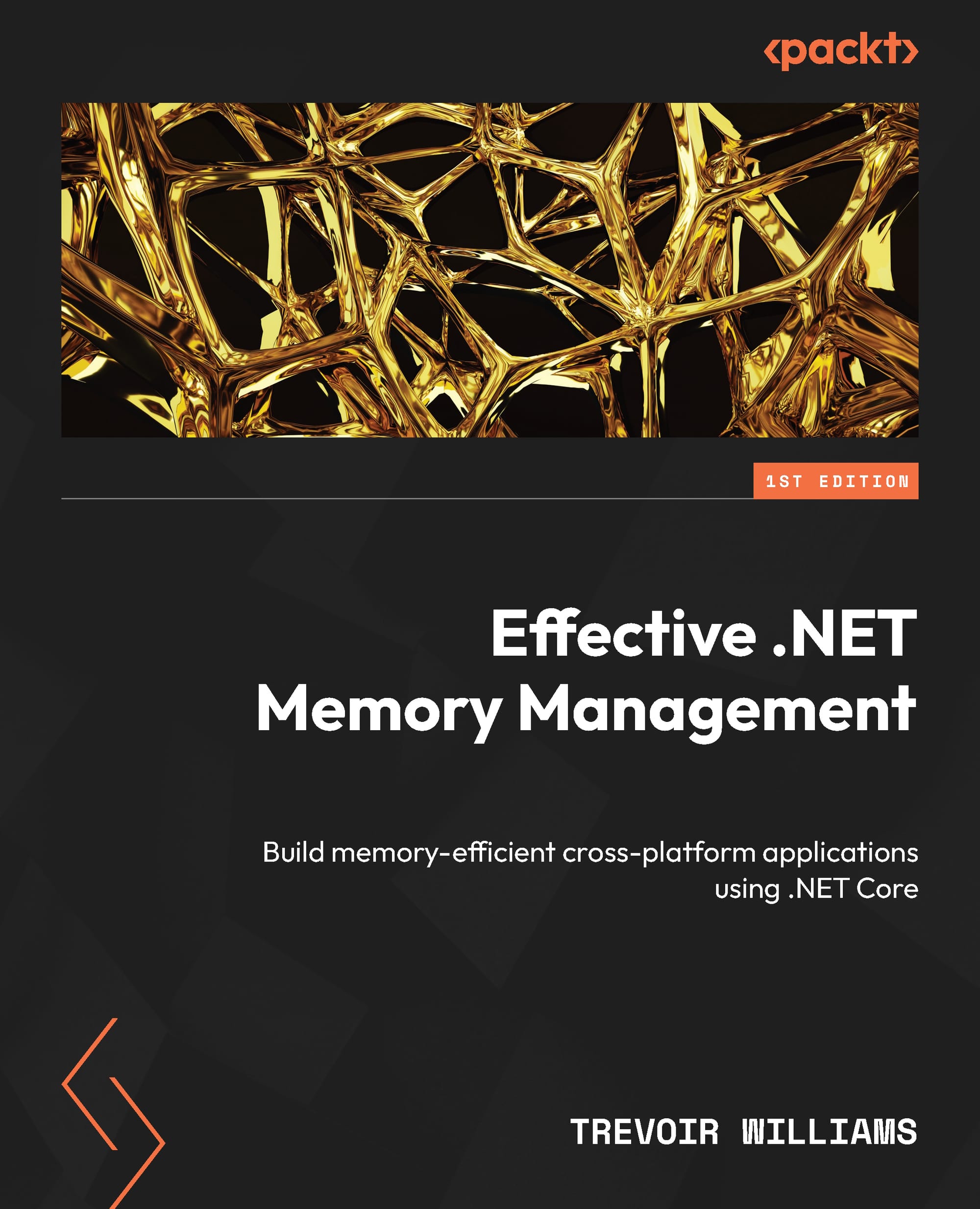
Effective .NET Memory Management, written by Trevoir Williams, is your essential guide to mastering the complexities of memory management in .NET programming. This comprehensive resource equates developers with the tools and techniques to build memory-efficient, high-performance applications.
The book delves into fundamental concepts like:
· Memory Allocation and Garbage Collection
· Memory profiling and Optimization Strategies
· Low-level programming with Unsafe Code
Through practical examples and best practices, you’ll learn how to prevent memory leaks, optimize resource usage, and enhance application scalability. Whether you’re developing desktop, web, or cloud-based applications, this book provides the insights you need to manage memory effectively and ensure your .NET applications run smoothly and efficiently.
Get your Effective .NET Memory Management copy today and take your .NET skills to the next level!
Member discussion