Classes and Structs in .NET: How They Affect Memory Allocation and Management
In .NET programming, choosing between classes and structs is a critical decision that can significantly impact how memory is allocated and managed in your applications. While both can be used to define custom data types, they behave very differently under the hood regarding memory management. This blog post explores the key differences between classes and structs, how they affect memory allocation and best practices for choosing the right one.
What Are Classes and Structs?
In .NET, classes and structs are the most commonly used building blocks for creating custom data types.
- Classes are reference types allocated on the heap, supporting inheritance, polymorphism, and encapsulation.
- Structs are value types allocated on the stack (in most cases) and are typically used for small, lightweight objects.
While both can contain fields, properties, methods, and constructors, their memory allocation and management behavior differ, leading to performance implications that developers must consider.
Key Differences Between Classes and Structs
The following table outlines the primary differences between classes and structs:
Aspect | Class | Struct |
---|---|---|
Type | Reference Type | Value Type |
Memory Allocation | Allocated on the heap | Allocated on the stack (except for fields of other objects) |
Memory Management | Managed by the Garbage Collector (GC) | Automatically deallocated when it goes out of scope |
Inheritance | Supports inheritance and polymorphism | Does not support inheritance |
Performance | Generally slower due to heap allocation and GC | Faster for small, short-lived objects due to stack allocation |
Default Constructor | Supports parameterless constructors | Cannot have an explicit parameterless constructor |
Mutability | Often mutable | Typically immutable |
Usage Scenario | Use for larger, complex objects or when behavior needs to be shared | Use for small, lightweight objects and data that doesn't change often |
Memory Allocation for Classes vs. Structs
Classes: Reference Types
When you create an instance of a class, memory for that instance is allocated to the heap. Since the heap is designed to store objects with unpredictable lifetimes, the Garbage Collector (GC) manages the lifecycle of objects allocated on the heap. The GC periodically scans the heap, identifies no longer referenced objects, and frees up the memory.
Example of class allocation:
public class Car
{
public string Make { get; set; }
public string Model { get; set; }
}
Car car1 = new Car(); // Allocated on the heap
In this example, the car1
object is allocated on the heap. The variable car1
is stored on the stack, but it holds a reference to the object on the heap.
Because classes are reference types, when you pass a class instance to a method, you pass a reference to the original object. Changes made to the object inside the process will affect the original object.
Structs: Value Types
On the other hand, when you create an instance of a struct, memory for that instance is allocated on the stack (in most cases). The stack is a much smaller and faster memory region, ideal for short-lived, lightweight data types like structs. Unlike the heap, memory on the stack is automatically reclaimed when it goes out of scope, meaning the GC does not need to manage it.
Example of struct allocation:
public struct Point
{
public int X { get; set; }
public int Y { get; set; }
}
Point p1 = new Point(); // Allocated on the stack
In this example, p1
is a value type allocated directly on the stack. When you pass a struct to a method, a copy of the struct is passed, meaning changes to the copy won’t affect the original.
Best Practices for Choosing Between Classes and Structs
Now that you understand the key differences between classes and structs and how they affect memory management let's look at some best practices for choosing the right type:
1. Use Classes for Larger, More Complex Objects
- If your data type represents a larger, more complex object with significant behaviors (such as methods and business logic) and has an unpredictable lifetime, use a class.
- Classes are better suited for objects that require inheritance or polymorphism.
2. Use Structs for Lightweight, Simple Data Types
- Structs are best used for small, lightweight data types that don’t require inheritance or complex behavior. They are typically used for modeling simple data structures such as coordinates (e.g.,
Point
) or dimensions (e.g.,Size
). - Example use case: Structs are excellent for modeling immutable data that doesn’t change frequently, as they are cheaper to copy and don’t require GC intervention.
3. Consider the Performance Implications
- For performance-critical applications, prefer structs when dealing with small, short-lived objects, as they avoid heap allocations and the overhead associated with garbage collection.
- However, be cautious with large structs, as copying them can lead to poor performance due to their value-type nature (copies are made whenever passed to methods).
4. Use Structs for Immutability
- Structs are often used for immutable objects—objects that, once created, cannot be modified. This makes them ideal for data types where you don’t expect the internal data to change.
- Immutability improves safety, especially in multi-threaded environments where concurrent modifications could cause unexpected behavior.
5. Avoid Structs for Large Data
- Avoid using structs for large or complex objects. Since structs are copied by value, large structs can incur significant performance costs due to copying. Using a reference type (class) is more appropriate in these cases.
Conclusion
Choosing between a class or a struct in .NET has significant implications for memory allocation and management. As reference types, classes offer more flexibility with features like inheritance but incur the overhead of heap allocation and garbage collection. Structs, as value types, offer performance advantages using the stack, but they are more suited for small, lightweight, and immutable data types.
By understanding the key differences and following best practices, you can make informed decisions to optimize the memory usage and performance of your .NET applications.
Ready to dive deeper? Get your copy of Effective .NET Memory Management today!
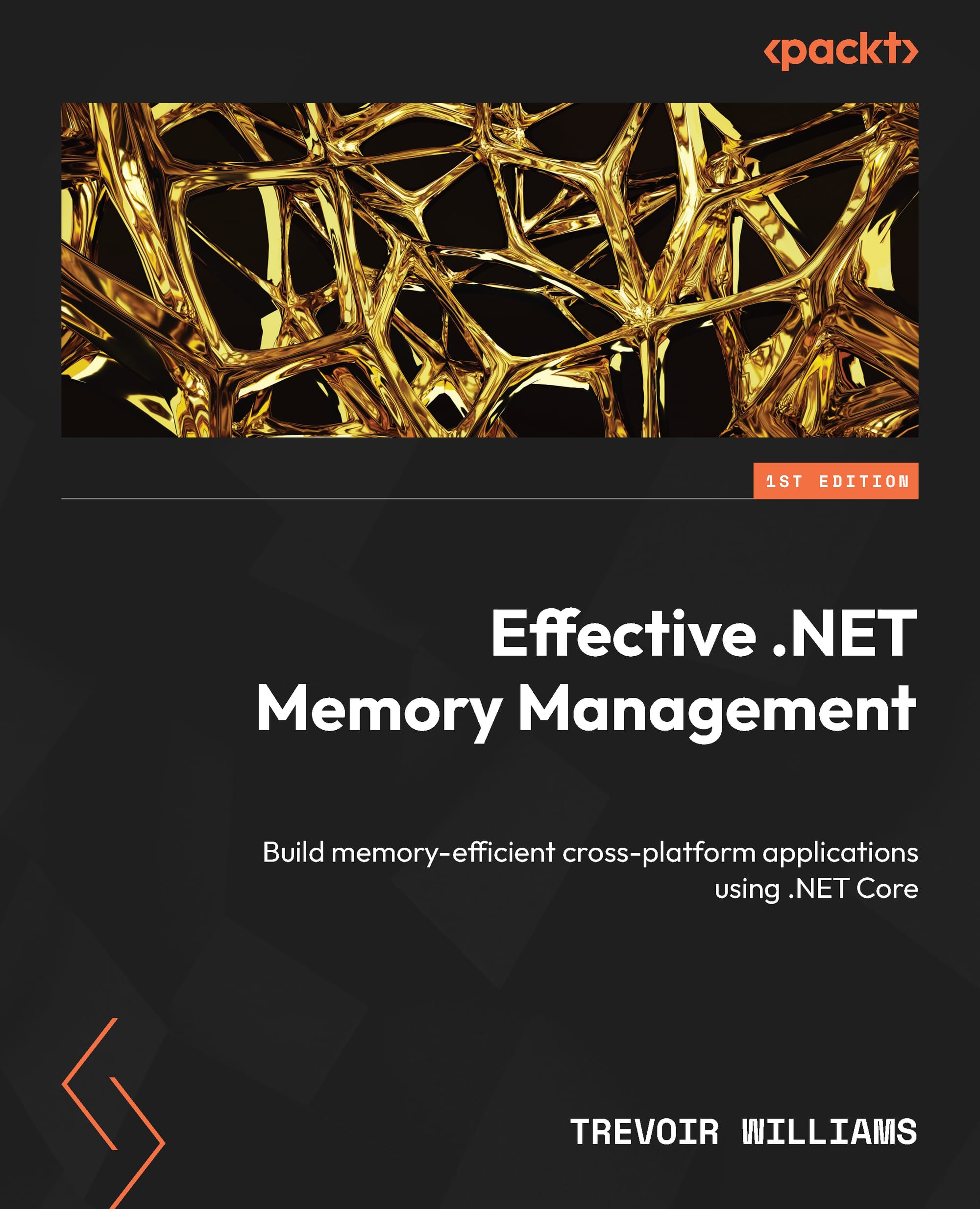
Member discussion