Best Practices for Managing Object Lifetimes in .NET
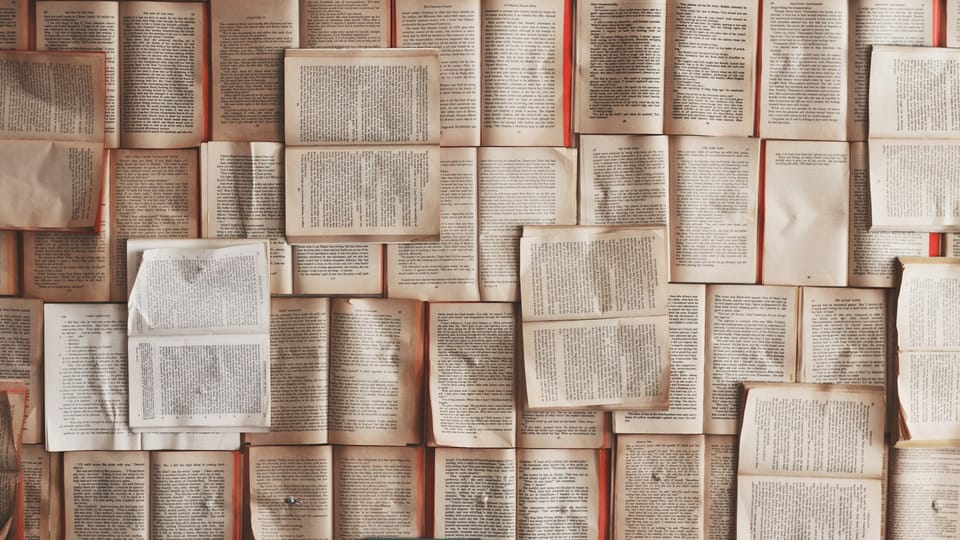
Managing object lifetimes is a critical aspect of memory management in .NET applications. Properly handling how long objects remain in memory can significantly impact the performance and efficiency of your software.
Continuing our previous discussion on Understanding Objects and How They Are Created in .NET, where we explored object creation and memory allocation fundamentals, this article delves deeper into the best practices for managing object lifetimes in .NET. Building on the concepts of object creation, we will now focus on effectively managing the lifespan of objects to optimize performance and ensure efficient resource usage in your applications.
In .NET, an object's lifetime is the period during which it is allocated in memory and accessible by your application. The Common Language Runtime (CLR) manages memory allocation for objects. However, developers must still be mindful of how long objects live and when they should be disposed of to avoid memory leaks and other performance issues.
1. Use the IDisposable
Interface
One of the most essential practices in managing object lifetimes is implementing the IDisposable
interface for objects that manage unmanaged resources, such as file handles, database connections, or network streams. The IDisposable
interface provides a Dispose
method, which should be called to release these resources when they are no longer needed.
Here's a code snippet from the book that demonstrates the IDisposable
pattern:
public class UnitOfWork : IUnitOfWork, IDisposable
{
private readonly ApplicationDbContext _context;
private bool _disposed = false;
public UnitOfWork(ApplicationDbContext context)
{
_context = context;
}
public void Dispose()
{
Dispose(true);
GC.SuppressFinalize(this);
}
protected virtual void Dispose(bool disposing)
{
if (!_disposed)
{
if (disposing)
{
// Dispose managed resources
_context.Dispose();
}
// Dispose unmanaged resources here if needed
_disposed = true;
}
}
public async Task Save()
{
await _context.SaveChangesAsync();
}
}
In this example, the Dispose
method ensures that the ApplicationDbContext
is appropriately disposed of when the UnitOfWork
is no longer used. This pattern helps prevent memory leaks by explicitly releasing resources at the end of an object's lifecycle.
2. Leverage the using
Statement
The using
statement in C# is a convenient way to manage object lifetimes for objects that implement IDisposable
. It automatically calls the Dispose
method on the object when it goes out of scope, ensuring that resources are released promptly.
using (var connection = new SqlConnection(connectionString))
{
connection.Open();
// Use the connection here
} // Dispose is automatically called when the block is exited
Using the using
statement helps encapsulate resource management, making your code cleaner and reducing the risk of forgetting to dispose of objects.
3. Minimize the Lifetime of Large Objects
Large objects in .NET (those over 85,000 bytes) are allocated in the Large Object Heap (LOH). Managing the lifetimes of large objects is crucial because the Garbage Collector does not compact the LOH, which can lead to fragmentation and inefficient memory use.
If possible, avoid holding large objects in memory longer than necessary. Consider breaking down large objects into smaller, more manageable pieces that the CLR can handle more efficiently.
4. Use Weak References for Short-Lived Data
When dealing with short-lived data, consider using weak references. A weak reference allows the Garbage Collector to collect an object while still allowing it to be accessed if it's still in memory. This is useful for caching scenarios where you want to keep data around only if it is convenient.
var cache = new WeakReference(myObject);
if (cache.IsAlive)
{
var obj = cache.Target as MyObjectType;
// Use the object
}
else
{
// Recreate the object
}
Using weak references can help prevent unnecessary memory retention, especially when objects can be recreated if needed.
5. Employ Object Pooling
Object pooling is a technique in which objects are reused rather than repeatedly created and destroyed. This is particularly useful in high-performance applications where object creation overhead can impact performance.
.NET Core provides a built-in ObjectPool
class that can be used to manage object pooling:
var pool = new DefaultObjectPool<MyObject>(new DefaultPooledObjectPolicy<MyObject>());
var obj = pool.Get();
// Use the object
pool.Return(obj); // Return the object to the pool for reuse
Object pooling can significantly reduce memory allocations and garbage collection overhead, making your application more efficient.
Conclusion
Managing object lifetimes effectively is crucial to building performant and reliable .NET applications. By implementing these best practices—using the IDisposable
pattern, leveraging the using
statement, minimizing the lifetime of large objects, using weak references, and employing object pooling—you can ensure that your applications make the best use of memory and resources.
For a deeper dive into these topics, I highly recommend picking up a copy of Effective .NET Memory Management by Trevoir Williams. This book provides comprehensive coverage of memory management in .NET and practical tips and techniques that every developer should know.
Ready to master memory management in .NET? Get your copy of Effective .NET Memory Management today, and take your skills to the next level!
Member discussion