Accessing Contiguous Memory in .NET Core: Best Practices and Techniques
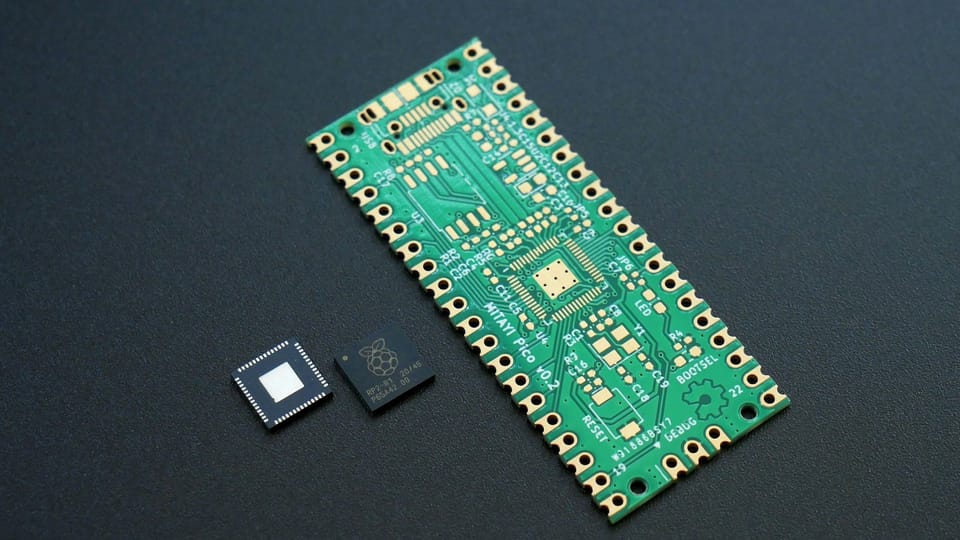
When writing high-performance .NET Core applications, understanding how to efficiently access memory is crucial. Memory access patterns can significantly affect the speed and efficiency of your code, mainly when dealing with large datasets or performance-critical applications. One important concept to master is accessing contiguous memory, which can help reduce cache misses, improve locality, and boost application performance.
This article will explore contiguous memory, why it's essential, and how you can access and manage it effectively in .NET Core.
What is Contiguous Memory?
Contiguous memory refers to a block of memory locations that are sequentially ordered. When data is stored in contiguous memory, it can be accessed more quickly because the CPU can efficiently load the data into its cache, reducing the need for costly memory fetch operations. Contiguous memory is often associated with arrays stored in a continuous block of memory. This means accessing elements in an array can be much faster than accessing elements in a more fragmented data structure like a linked list.
Why Contiguous Memory Matters
Accessing contiguous memory is important for several reasons:
- Cache Efficiency: Modern CPUs use caches to store frequently accessed data. When data is stored contiguously, the CPU can load larger chunks into the cache simultaneously, reducing the number of cache misses.
- Improved Performance: The hardware can optimize sequential access patterns, leading to faster read and write operations.
- Reduced Fragmentation: Contiguous memory allocation reduces the likelihood of memory fragmentation, which can occur when memory is allocated in scattered locations.
How to Access Contiguous Memory in .NET Core
In .NET Core, there are several ways to ensure that your data is stored contiguously in memory. Here are some of the most effective techniques:
1. Using Arrays
Arrays are the simplest and most common way to allocate contiguous memory in .NET. Since arrays are stored in a single, contiguous block of memory, they allow fast and efficient data access.
int[] numbers = new int[1000];
for (int i = 0; i < numbers.Length; i++)
{
numbers[i] = i * 2;
}
In this example, the numbers
array is stored in contiguous memory, making it easy to iterate and access the elements quickly.
2. Span and Memory
With .NET Core, Span<T>
and Memory<T>
are powerful tools for working with contiguous memory while avoiding unnecessary allocations.
- Span: A
Span<T>
represents a contiguous region of arbitrary memory. It can point to any piece of memory, including stack, heap, or unmanaged memory, and allows you to safely and efficiently manipulate memory.
Span<int> numbersSpan = stackalloc int[1000];
for (int i = 0; i < numbersSpan.Length; i++)
{
numbersSpan[i] = i * 2;
}
In this example, stackalloc
allocates a contiguous block of memory on the stack. The Span<int>
provides a safe and efficient way to access and manipulate that memory.
- Memory: Unlike
Span<T>
,Memory<T>
is not stack-only and can be used for asynchronous operations. It’s useful when working with contiguous memory across asynchronous boundaries.
Memory<int> numbersMemory = new int[1000];
for (int i = 0; i < numbersMemory.Length; i++)
{
numbersMemory.Span[i] = i * 2;
}
Memory<T>
is ideal for scenarios where you need to manipulate memory in a contiguous fashion, but the memory needs to survive beyond the current method scope.
3. Using Unsafe Code for Pointers
For advanced scenarios where performance is critical, you can use unsafe code to work directly with pointers. This allows you to manipulate memory locations directly but comes with risks and requires careful management.
unsafe
{
int[] numbers = new int[1000];
fixed (int* p = numbers)
{
for (int i = 0; i < 1000; i++)
{
*(p + i) = i * 2;
}
}
}
In this example, fixed
is used to pin the array in memory, and a pointer is used to directly access and modify the elements. While powerful, this approach requires a solid understanding of memory management and is typically reserved for performance-critical applications.
Best Practices for Working with Contiguous Memory
When working with contiguous memory in .NET Core, keep the following best practices in mind:
- Prefer Safe Alternatives: Use
Span<T>
andMemory<T>
when possible, as they provide safety and efficiency without the risks associated with unsafe code. - Minimize Allocations: Reducing the number of memory allocations can improve performance by minimizing garbage collection overhead.
- Profile Your Application: Use profiling tools to monitor memory usage and ensure that your memory access patterns are efficient.
- Avoid Unnecessary Copies: When working with large data sets, avoid unnecessary copying of data to reduce memory usage and improve performance.
Conclusion
Accessing contiguous memory in .NET Core is a key technique for improving the performance and efficiency of your applications. By understanding and utilizing tools like arrays, Span<T>
, Memory<T>
, and even unsafe code when necessary, you can ensure that your applications make the best use of available memory.
For a deeper dive into these techniques and more advanced memory management strategies, check out Effective .NET Memory Management by Trevoir Williams. This book provides a comprehensive guide to mastering memory management in .NET, making it an essential resource for any serious .NET developer.
Ready to optimize your .NET applications? Get your copy of Effective .NET Memory Management today and unlock the full potential of your .NET development skills!
Member discussion